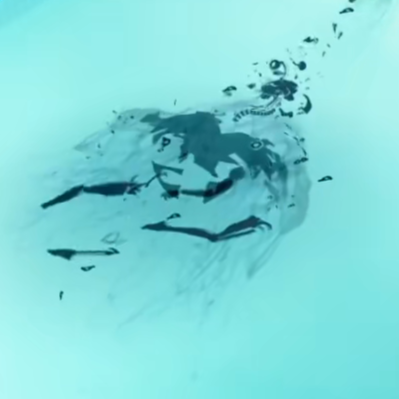
C++ 技巧1
virtual函数
虚函数的调用取决于指向或者引用的对象的类型,而不是指针或者引用自身的类型。
静态函数不可以声明为虚函数,同时也不能被const
和 volatile
关键字修饰。
构造函数不可以声明为虚函数。同时除了inline|explicit
之外,构造函数不允许使用其它任何关键字。
多态
本质上是,利用继承和虚函数实现多种不同的类调用同一个函数,此函数在不同的子类中有不同的实现,但函数名一样。
模板
模板函数
提供一种操作,但支持多种数据类型,可有效减少代码量,适用于多种数据进行同类型操作
template <typename T> |
声明中typename与class没有差别
自动推导数据类型时,必须推导出一致的数据类型才能使用
在没有确定类型参数的情况下,模板无法自动推导,此时模板没有确定的数据类型,那么模板无法使用,必须手动指定数据类型
普通函数调用时可以发生自动类型转换(隐式类型转换),但模板函数自动推导类型时无法隐式类型转换,只有指定类型后才可以
从某种程度上说,函数模板在指定类型后,与普通函数相差不大
对用规则
函数模板与普通函数都可以实现的情况下,优先使用普通函数
可以通过空模板参数来强制调用函数模板
函数模板可以重载
具体化函数模板
函数模板不能直接传入自定义类型或数组,可以通过具体化函数模板来解决
class person{ |
“学习模板不是为了写模板,而是为了熟练运用STL提供的模板”
类模板
创建一个数据类模板,此模板可以使用多个未定的数据类型,给其赋值时需要指定数据类型
template<class NameType, class AgeType = int> |
类模板无法自动推导类型,但可以有默认参数(即默认的数据类型)
类模板中的成员函数在调用时才生成
当类模板的对象作为函数的参数时,可以指定传入对象的数据类型,也可以将参数模板化,或将整个类模板化
类模板与继承
当子类继承的父类是一个类模板时,子类在声明的时候,要指定出父类中T的类型
如果不指定,编译器无法给子类分配内存
如果想灵活指定出父类中T的类型,子类也需变为类模板
文件读写
正常读取:
|
判断文件是否为空:
|
写入文件:
|
退出程序接口
exit(0) |
linux下的“按任意键继续”
This is a library conio.h
for linux. Just copy file and paste file conio.h
on /usr/include/
but don’t forget before you want copy paste on /usr/include/
you must open folder as ADMINISTRATOR
first !!
git clone https://github.com/zoelabbb/conio.h.git |
重启IDE
|
string一个用法(把字符串当做字符数组)
void speechManager::createPlayer() { |
遍历容器(使用auto)
for (auto it = vector.begin(); it != vector.end(); it ++){ |
特性
using
The modern cpp use more using
to define aliases of variables.
using byte = unsigned char; |
namespace
When a project was completed by many coders, naming conflicts in so many identifiers may be occurs.
Key word namespace
can define a namespace with a name or not. The same indentifiers can exist in different namespaces.
Using ::
access to a namespace.
Don’t use ;
at the end of namespace.
Increment and Decrement
int i = 1; |
Both
i++
and++i
will makei
plus 1, but when using them in aclass
,++i
is more efficienct.
Built-in operation function
|
Type convertion
There are three ways to convert:
(DestinationType)sourceData; |
if
/ switch
with initialization
Limit the scope of variables as much as possible.
cpp 17 introducedif
/switch
statements that allow variable initialization.
if (auto x{ std::cin.get() }; x >= 48 && x <= 57){ |
Range-based for
int a[]{ |
Function and Reference
Function
double my_sqrt(double x) { |
Result:
entering main |
To iterate is human, to recurse divine.
To recieve multiple data, usinginitializer_list
to send parameters to function.
e.g.
double sum(std::initializer_list<double> ld) { |
The result:
10 |
Inline function
Insert the inline
keyword in front of the function defination, which is recommanded.
When the resouces of codes in a function are less than calling the function, using inline function can increase spending saving.
Inlining is at the cost of code bloat(copying), and only saves the overhead of function calls, thereby improving the execution efficiency of functions.
Inline should not be used in the following situation:
- If the code in the function body is relatively long, making inlining will lead to higher memory consumption costs.
- If there is a loop int the function body, the time to execute the code in the function body is greater than the overhead of the function call.
Default parameter
Provide the default parameters.
When calling the function, it can automatically use the default parameters without your actual parameters.
Function Template
Function templates implement parameterization of data types.
It can use unknown data types as parameters. As long as the type of data meets the requirements defined by the function template, the function template can be called with these types of data as arguments.
template <typename T> T my_min(T a, T b) { return a < b ? a : b; } |
Lambda Function
int add(int x, int y) { return x + y; } |
Lambda function is a function that can capture the variables in a scope autometically.[]
means the capture list.
There are several commonly used forms of capture lists:
Forms | Meaning |
---|---|
[x] |
Capture the variable x by value passing |
[=] |
Capture all variables in the parent scope by value passing |
[&x] |
Capture the variable x by reference passsing |
[&] |
Capture all variables in the parent scope by reference passing |
[=,&x,&y] |
Capture the variables x and y by reference passing and the rest by value passing |
[&,x] |
Capture the variable x by value passing and the rest by reference passing |
Lambda function can have the real parameters.
Reference
Left-valued reference
The reference is a key point and difficulty.
A reference is an alias of the referenced object. The two are essentially the same object, but they are displayed as different names and types.
T& r = t; |
Where T
is a data type, &
is an operator, r
is reference name and t
is a variable of type T
.
t
cannot be constant or right value expression.r
must be initialized when defined.
This is excatly where the reference is diferent from the pointer, that is, there is no empty reference.
References cannot exist independently, but must be attached to the referenced variable, so it does not take up memory space.
If the reference is a named variable, such a reference is called left-valued reference (in cpp98).
int a = 2; |
The result:
a: 2 |
It can be seen that the left value reference is only an alias of the variable, so all operations on the lvalue reference are equivalent to the operation on the variable itself.
Supplementary explanation:
- Any variable can be reference, such as pointer.
int m = 3; |
Reference is not variable, so you cannot reference a reference! And at the same time, pointers cannot point to a reference.
- Pointers can be
nullptr
orvoid
type, but references cannot.
int m = 3; |
- Cannot build an array of reference.
- Constant lvalue reference can be initialized by lvalue, constant lvalue and rvalue.
int x = 1; |
The left reference of the constant is usually used as a formal parameter of the function. At this time, the corresponding actual parameter cannot be modified inside the function through this reference to achieve the purpose of protecting the actual parameter.
Rvalue reference, move()
and Move semantics
template <typename T> void my_swap(T &a, T &b) { |
Actually it is the swap()
in cpp STL.
The cpp STL also provides array exchange in the form of overloading.
template <class T, std::size_t N> void my_swap(T (&a)[N], T (&b)[N]) { |
attribute
在C++中,__attribute__
是一种GCC编译器提供的扩展语法,用于在函数、变量、类型等声明中添加附加属性。它的语法形式为__attribute__((属性列表))
。
一些常见的属性和它们的说明:
__attribute__((aligned(n)))
:指定变量或结构体的对齐方式为n字节。例如,__attribute__((aligned(4))) int x;
将x的对齐方式设置为4字节。__attribute__((packed))
:告诉编译器取消结构体的对齐,即以最小的字节对齐结构体成员。这在需要与外部系统或文件进行二进制数据交换时非常有用。__attribute__((noreturn))
:用于标记函数不会返回。例如,__attribute__((noreturn)) void error();
表示函数error
不会返回。__attribute__((unused))
:告诉编译器该变量可能未使用,可以抑制未使用变量的警告。例如,int x __attribute__((unused));
告诉编译器x可能未使用。__attribute__((deprecated))
:用于标记函数、变量或类型已被弃用。当使用被标记为弃用的元素时,编译器会发出警告。__attribute__((constructor))
和__attribute__((destructor))
:用于定义在程序启动前或结束后自动执行的函数。constructor
属性用于在main
函数执行之前自动调用的函数上,而destructor
属性用于在程序结束前自动调用的函数上。
__attribute__
是GCC的扩展特性,不是C++标准的一部分,因此在使用时应该注意可移植性。
declspec
Deduce the type of statements when compiling. It can be used to define a variable.
int a = 0; |
declspec
用以推测表达式结果的类型,返回此类型;而__declspec(dllimport)
是 Microsoft Visual C++ 编译器的一个扩展,用于在编译动态链接库(DLL)时指示一个函数或变量是从外部DLL中引入的。
当你在一个程序中需要使用从外部DLL中导出的函数或变量时,你可以使用 declspec(dllimport)
关键字来告诉编译器这个函数或变量是从其他DLL中引入的,而不是在当前代码中定义的。这样,编译器在编译时会生成适当的代码,以便正确地链接到外部DLL中的内容。
为了提高代码的可读性,请为 __declspec(dllimport)
定义宏,并使用此宏来声明导入的每个符号:
|
虽然在函数声明中使用 __declspec(dllimport)
是可选的,但如果你使用此关键字,编译器会生成更高效的代码。 不过,必须对导入的可执行文件使用 **__declspec(dllimport)
**,以访问 DLL 的公共数据符号和对象。 请注意,DLL 的用户仍需要与导入库链接。
可以对 DLL 和客户端应用程序使用相同的头文件。 为此,请使用特殊的预处理器符号来指示是生成 DLL 还是生成客户端应用程序。 例如:
|
Other
explicit
关键字可以帮助你在类的构造函数中明确地指定类型转换的行为,从而提高代码的可读性和安全性。
optional
在C++中,std::optional
是C++17标准引入的一个类模板,用于表示一个可能存在或可能不存在的值。它提供了一种更安全、更语义清晰的方式来处理可能缺失的值,而不需要使用传统的空指针或特殊值。std::optional
可以看作是一种对可能的值进行了封装的容器,它要么包含一个有效的值,要么为空(不包含任何值)。
使用std::optional
的主要好处包括:
避免空指针异常:传统的空指针可能在访问时引发未定义行为,而
std::optional
通过类型系统和成员函数来明确指示值的存在或不存在,从而避免了潜在的运行时错误。更好的语义表达:
std::optional
能够更清晰地表达一个值是可选的,而不需要通过注释或命名来传达这种信息。避免特殊值:使用
std::optional
可以避免使用特殊值(例如-1、0或空字符串)来表示缺失的值,从而增加了代码的可读性和维护性。优雅的值处理:
std::optional
提供了一些成员函数,如has_value()
、value()
和value_or()
,使得对可能存在的值进行访问和处理更加优雅和安全。
以下是一个简单的示例,展示了如何在C++中使用std::optional
:
|
在这个示例中,std::optional
被用来包装除法操作的结果,以便在可能出现除零错误时能够明确地表示值的缺失。
- 本文标题:C Plus Plus - Skill
- 创建时间:2022-05-25 17:34:01
- 本文链接:2022/05/25/note/Programming/Language/cpp-4/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!