Project Manager
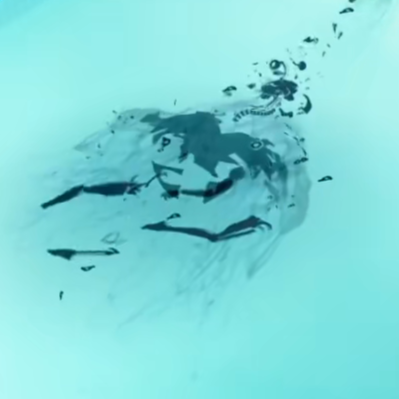
需求:
- 可以创建以 CMake + make 为构建工具的 C++项目
- 可以添加或删除 C++ 类,自动生成
.h
和.cpp
文件,并补全必要代码 - 可以使用命令进行构建和运行项目
- 可以读取配置文件信息,如果没有,会初始化创建一个配置文件,配置文件信息包括:项目的路径,该路径中的所有项目,指定当前项目
命令行参数及作用
long arg | arg | do |
---|---|---|
--list |
-l |
show the whole inforamtion |
--createproject |
-c |
create project |
--delproject |
-d |
delete a prject |
--addclass |
-a |
add class |
--delclass |
none |
delete a class |
--build |
-b |
build without run |
--run |
-r |
build and run |
--setproject |
-s |
set the current project |
--setpath |
none |
set the project path |
--help |
-h |
show help information |
项目地址
https://gitee.com/sential/projectmanager
安装脚本
|
开发过程中学到的东西
命令行参数
使用getopt()
函数,原型为:int getopt(int argc, char *const *argv, const char *shortopts)
或如果需要长参数,使用getopt_long()函数,原型为: int getopt_long(int argc, char *const *argv, const char *shortopts, const struct option *longopts, int *longind)
使用方法是先创建三个参数,分别为命令行参数opt
用以判定参数到底是哪一个,参数索引option_index
,和长参数结构体数组本身long_options[]
其中long_options[]
的option
类型的结构的,原型为:
struct option { |
创建参数变量:
int opt, option_index = 0; |
然后创建一个获取参数的循环,使用函数getopt_long
不断的获取参数,用switch
判断参数是哪个,然后执行相应的动作
while ((opt = getopt_long(argc, argv, "lbra:c:hd:s:D:S:", long_options, |
打印彩色字符
在输出流的字符前加上\033[1m
,可让字符高亮显示,[
后的数字替换成其他可实现更多的效果:
code | 效果 |
---|---|
1 |
让输出的字符高亮显式 |
3 |
输出斜体字 |
4 |
给输出的字符加上下划线 |
5 |
让输出的字符闪烁显式 |
7 |
设置反显效果,即把背景色和字体颜色反过来显示 |
30 |
表示黑色 |
31 |
表示红色 |
32 |
表示绿色 |
33 |
表示黄色 |
34 |
表示蓝色 |
35 |
表示紫色 |
36 |
表示浅蓝色 |
37 |
表示灰色 |
40 |
表示背景为黑色 |
41 |
表示背景为红色 |
42 |
表示背景为绿色 |
43 |
表示背景为黄色 |
44 |
表示背景为蓝色 |
45 |
表示背景为紫色 |
46 |
表示背景为浅蓝色 |
47 |
表示背景为灰白色 |
0 |
清除所有格式 |
输出的格式像是一个状态机,输出流加入\033[31m
后的所有字符都会是红色,取消这个效果要加上\033[0m
,然后再进行其他的操作。
Use bash by String
std::string cmd = |
- 本文标题:Project Manager
- 创建时间:2023-05-25 17:34:01
- 本文链接:2023/05/25/note/Programming/Projects/projectmanager/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!
显示评论